A simple PHP datagrid control for CodeIgniter framework with Bootstrap. SmartGrid focus on data display than data manipulation. We are starting with limited features to make the code very simple and robust, yet we will be adding more feature on the go. The code is very simple and well documented, which make it easy for customization.
Requirements
Installation
- Download the source files
- Copy the folder and files
application/libraries/SmartGrid/
toapplication/libraries/
folder of your CodeIgniter installation - That's it! Start using with the examples
Downloads
Features
- Simple datagrid for data display
- Accepts both MySQL query and array data
- Automatic Pagination
- Uses Twitter Bootstrap for styling, so easy to style with any bootstrap template
- No extra css or js files to include
Limitations
- Add, Edit, Del, Themes, Export, Search and Sort are not supported
- Search, Sort, Themes, Export features are on pipeline
- Database support: MySQL only for now
- Language support: English only for now
- Ajax is not support now
Screenshots
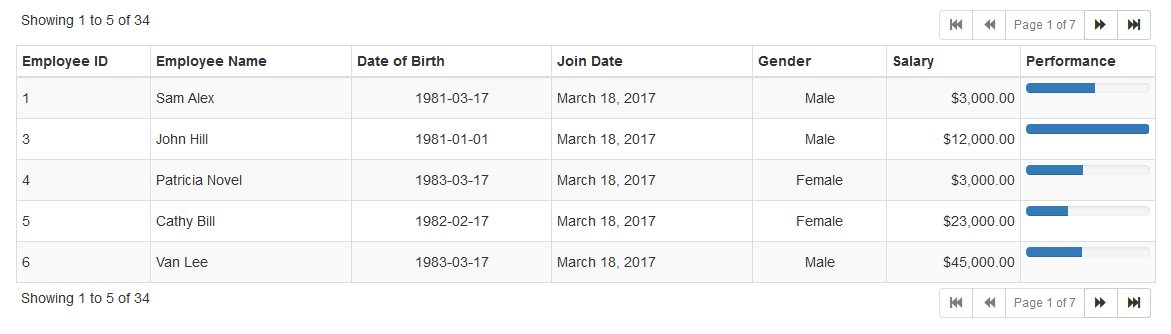
Example usage
on your controller:// Load the SmartGrid Library $this->load->library('SmartGrid/Smartgrid'); // MySQL Query to get data $sql = "SELECT * FROM employee"; // Column settings $columns = array("employee_id"=>array("header"=>"Employee ID", "type"=>"label"), "employee_name"=>array("header"=>"Name", "type"=>"label"), "employee_designation"=>array("header"=>"Designation", "type"=>"label") ); // Set the grid $this->smartgrid->set_grid($sql, $columns); // Render the grid and assign to data array, so it can be print to on the view $data['grid_html'] = $this->smartgrid->render_grid(); // Load view $this->load->view('example_smartgrid', $data);and then, on your view:
<!-- For styling, refer the bootstrap from CDN or from your server. Ignore this if you already have included in main view -->and on the same view:
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css">
// Print the grid html echo $grid_html;
Detailed description of usage
Let's break the above usage to get more idea on what is happening.First thing we do is load the SmartGrid library to the controller, the following line will do that pretty well.
$this->load->library('SmartGrid/Smartgrid');Now we have the SmartGrid library loaded on our controller, we will be able to call the functions in it.
1.First we have to initialize SmartGrid with datasource, column details and optionally with extra configuration. SmartGrid has 'set_grid' function for that,
$this->smartgrid->set_grid($sql_or_array, $columns, $config);set_grid function takes 3 parameters while the last two are optional.
$sql_or_array
: You can pass a MySQl query or a multi-dimensional array with data.
$columns
: The details of the columns to be shown on the grid, if you set the 'auto_generate_columns' to 'true'
it will automatically assigned.
$config
: The configuration settings for SmartGrid. This is optional
2. Now we will be able to get the html of the datagrid by calling 'render_grid'
$data['grid_html'] = $this->smartgrid->render_grid();and you can pass the '$data' array to your view and will be able to print it there
echo $grid_html;If you are not seeing a stylish grid you may have missed to include bootstrap correctly.
Configuration Parameters
Parameter Name | Description | Default Value |
---|---|---|
auto_generate_columns |
Autogenerate columns based on result set | false |
paging_enabled |
Enable/Disable paging | true |
toolbar_position |
Paging toolbar position. Allowed values top/bottom/both/none | 'both' |
page_size |
Maximum rows per page | 10 |
grid_name |
Grid name, used to identify grid if there are multiple grids on same page | 'sg_1' |
$config = array("page_size"=> 5, "grid_name"=> "sg_1", "toolbar_position"=> 'both');To assign the configuration you can use any of the following methods
While loading the SmartGrid Library, pass
$config
as a second parameter
$this->load->library('SmartGrid/Smartgrid', $config);Call the
set_configs
function directly, it should be called before set_grid
to take in effect
$this->smartgrid->set_configs($config);While calling the
set_grid
, pass $config
as a third parameter
$this->smartgrid->set_grid($sql, $columns, $config);
Column Types
label | Displays the field values as it is. | |||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
link | Displays the anchor link, where you can customize the url based on the field values.
Extra Parameters:
|
|||||||||||||||
date | Convert and displays the date formated as specified.
Extra Parameters:
|
|||||||||||||||
relativedate | Calculates and displays the relative date based on current date | |||||||||||||||
image | Displays the date formated as specified.
Extra Parameters:
|
|||||||||||||||
custom |
Substitute pseudo-variables in the field value with any of the values in that row.
Ex: 'Hello {employee_name}' in the field value will display by replacing 'employee_name' with the real data in that row. Uses CodeIgniter's Template Parser Class |
|||||||||||||||
money | Displays the money as specified. Uses PHP number_format function.
Extra Parameters:
|
|||||||||||||||
enum | Displays the data from an array source provided, assumes the field value as the key.
If the key not found, displays the field value itself.
Extra Parameters:
|
|||||||||||||||
html | Displays HTML by converting field value to HTML entities. Uses PHP htmlentities function. | |||||||||||||||
password/mask | Displays mask characters instead of field value, but shows same string length.
Extra Parameters:
|
|||||||||||||||
progressbar | Displays progressbar based on the field value. Assumes the field value is a percentage value.
Extra Parameters:
|
$columns = array( "employee_id"=>array("header"=>"Employee ID", "type"=>"label", "align"=>"left", "width"=>"100px"), "employee_name"=>array("header"=>"Employee Name", "type"=>"label", "align"=>"left", "width"=>"150px"), "employee_dob"=>array("header"=>"Date of Birth", "type"=>"date", "align"=>"center", "width"=>"150px", "date_format"=>"Y-m-d", "date_format_from"=>"Y-m-d H:i:s"), "employee_join_date"=>array("header"=>"Join Date", "type"=>"relativedate", "align"=>"left", "width"=>"150px"), "employee_gender"=>array("header"=>"Gender", "type"=>"enum", "source"=>$gender_list, "align"=>"center", "width"=>"100px"), "employee_salary"=>array("header"=>"Salary", "type"=>"money", "sign"=>"$", "align"=>"right", "width"=>"100px"), "performance_index"=>array("header"=>"Performance", "type"=>"progressbar", "align"=>"center", "width"=>"100px"), "employee_img_url"=>array("header"=>"Image", "type"=>"image", "align"=>"center", "width"=>"50px", "image_width"=>"50px"), );And it should passed to
set_grid
function
$this->smartgrid->set_grid($sql, $columns);